이전강의 보러가기.
2022.06.16 - [IT] - [Nomad Coders] TypeScript로 블록체인 만들기 #4 정리
[Nomad Coders] TypeScript로 블록체인 만들기 #4 정리
TS to JS 코드 연습 #4 CLASSES AND INTERFACES #4.0 Classes ts에서 object oritented 구현 ts에서 class 만들기 class Player { constructor ( private firstName:string, private lastName:string, public nick..
ella-devblog.tistory.com
#5 TYPESCRIPT BLOCKCHAIN
#5.0 Introduction
#5.1 Targets
#Create Typescript Project in VSCode
Visual Studio Code에서 타입스크립트 프로젝트를 생성해보자.
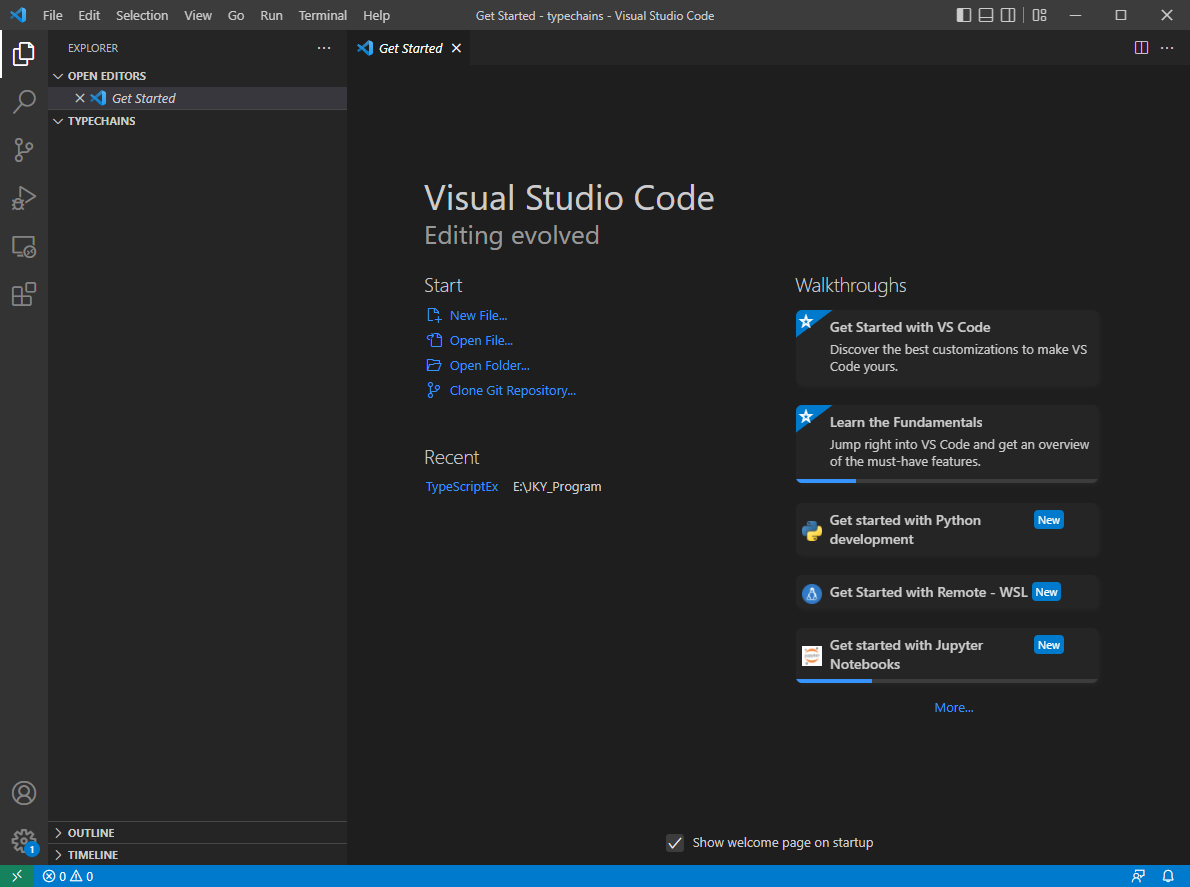
1. VS Code 에서 폴더를 연다. (folder name : typechains)
2. terminal을 열고 [>npm init -y] 를 입력한다.
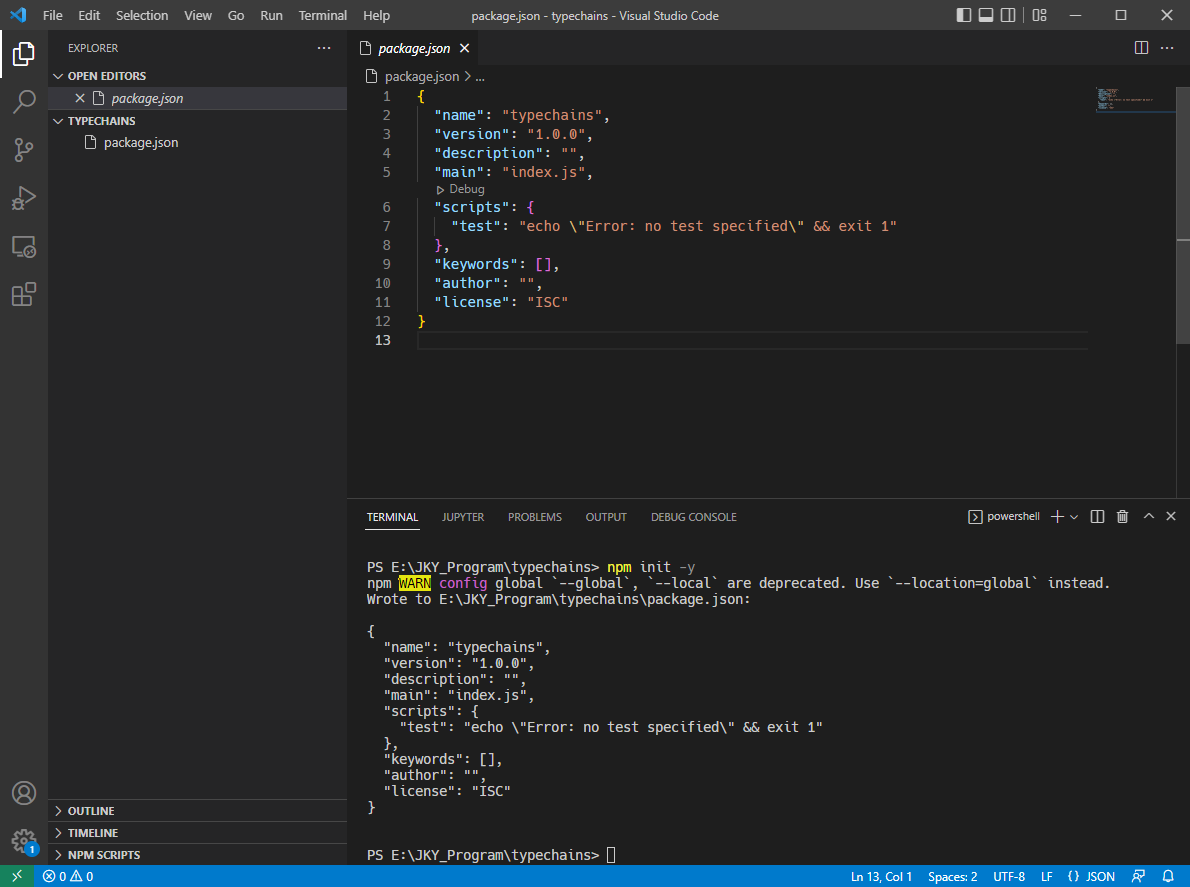
-> 그럼 package.json 파일이 생성된다.
3. package.json 파일을 수정해주고,
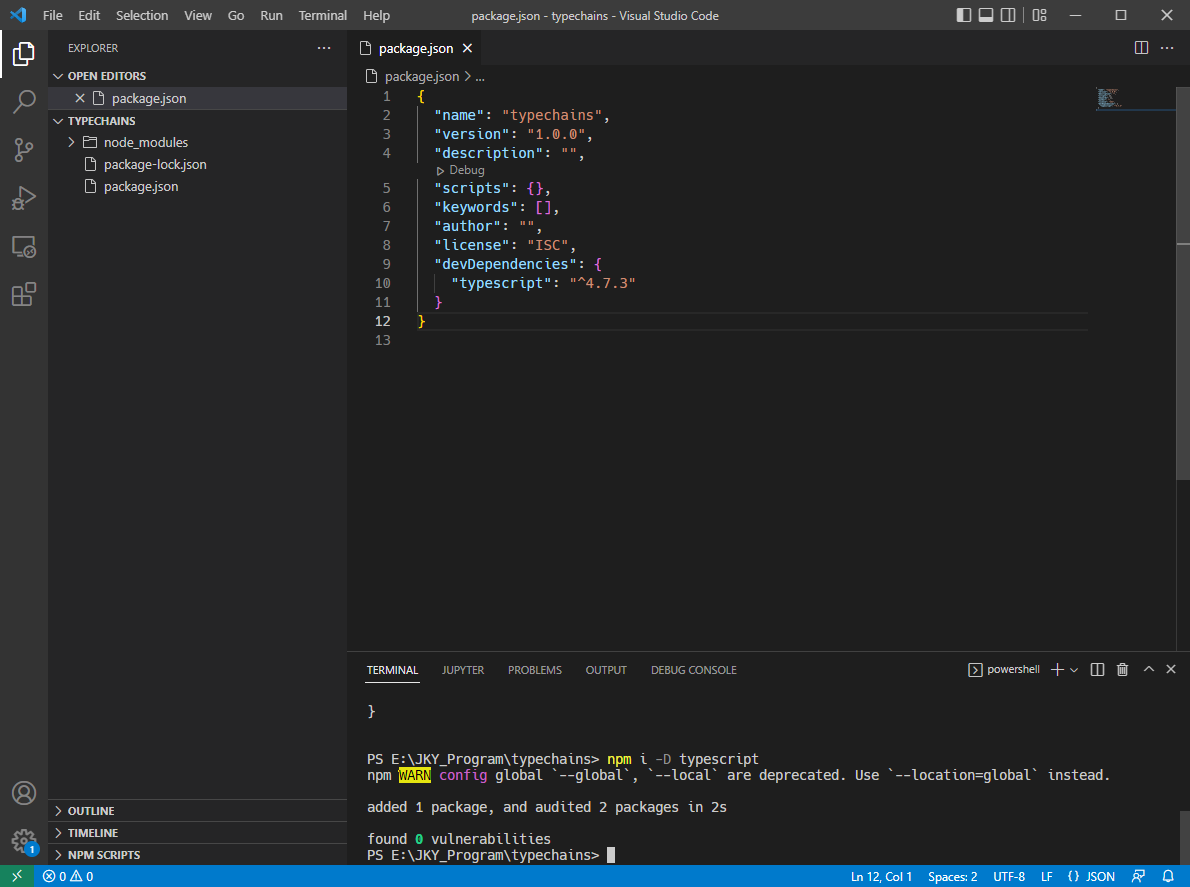
4. terminal에서 [>npm I -D typescript]를 입력해서 ts 설치해준다.
-D를 입력함으로써, ts가 defDependencies에 설치됨.
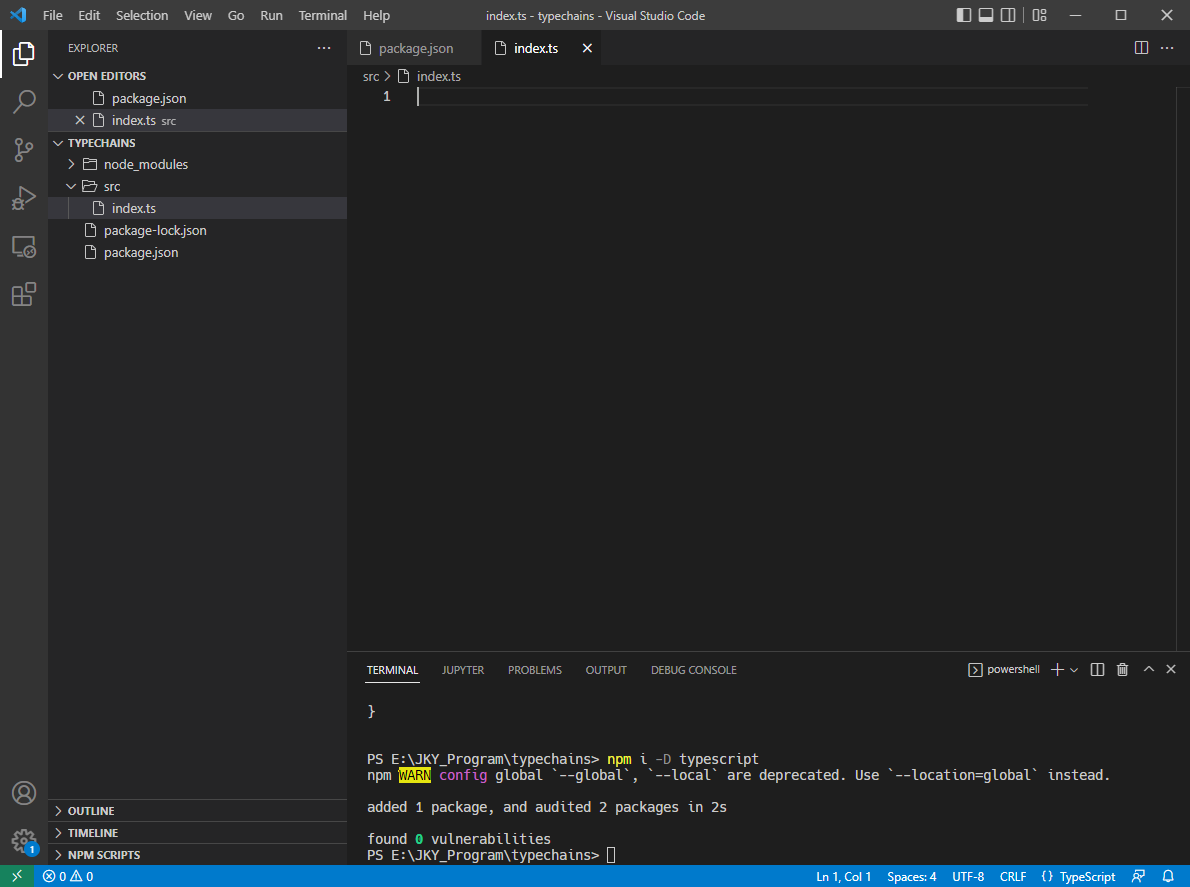
5. create new folder -> src, create new file -> index.ts
[index.ts]
const hello = () => "hi";
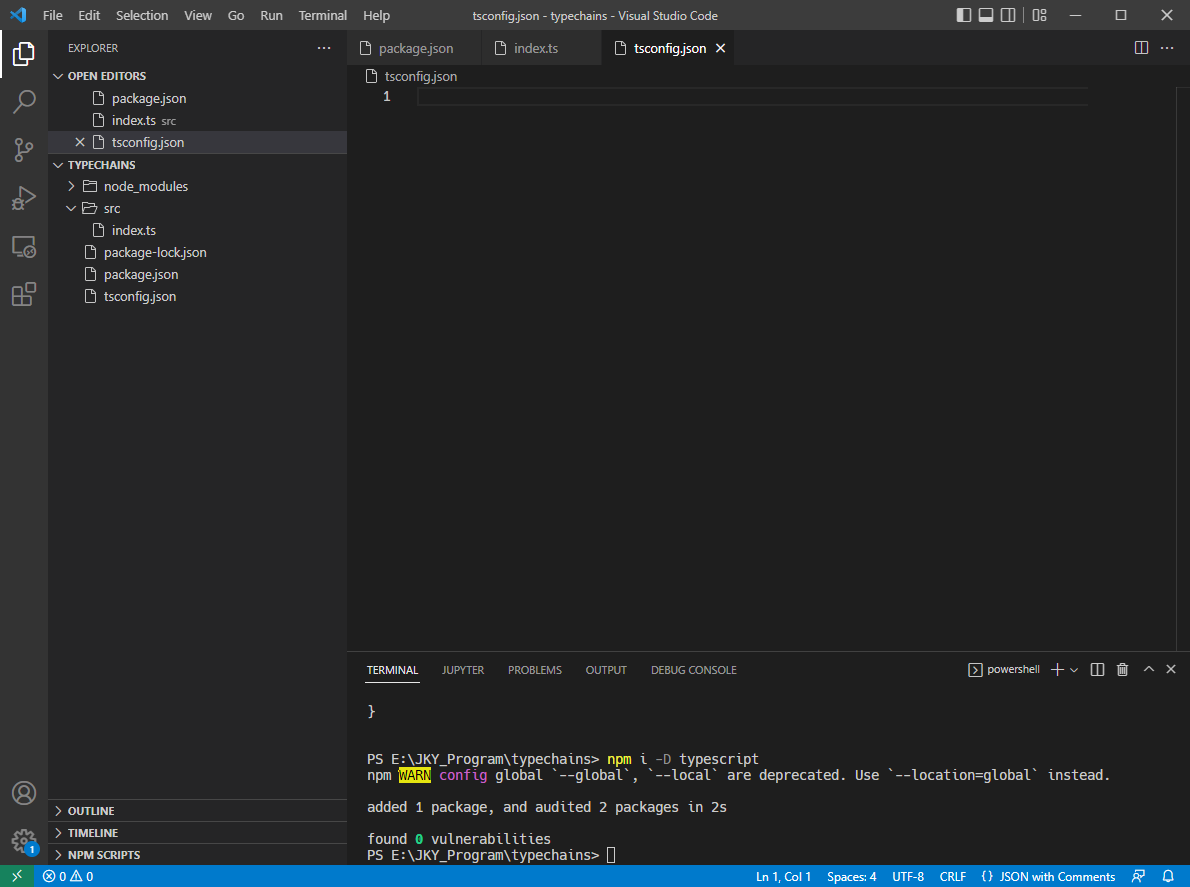
6. create new file -> tsconfig.json
tsconfig.json 파일의 이름은 무조건 tsconfig.json이어야 함.
include 배열에는 우리가 js로 컴파일하고 싶은 모든 directory를 넣어줘야함.
[tsconfig.json]
{
"include": ["src"],
"compilerOptions": {
"outDir": "build",
"target": "ES6",
"moduleResolution": "node"
}
}
컴파일 옵션에 outDir만 넣으면 build 폴더 생성이 안되서 댓글보고 위와같이 수정함.
"target" -> js 버전 설정
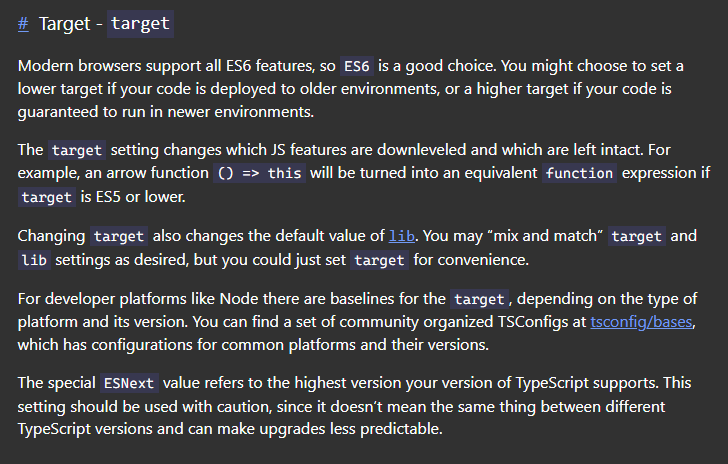
7. package.json 파일을 다시 수정해준다.
[package.json]
{
"name": "typechains",
"version": "1.0.0",
"description": "",
"scripts": {
"build": "tsc"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"typescript": "^4.7.3"
}
}
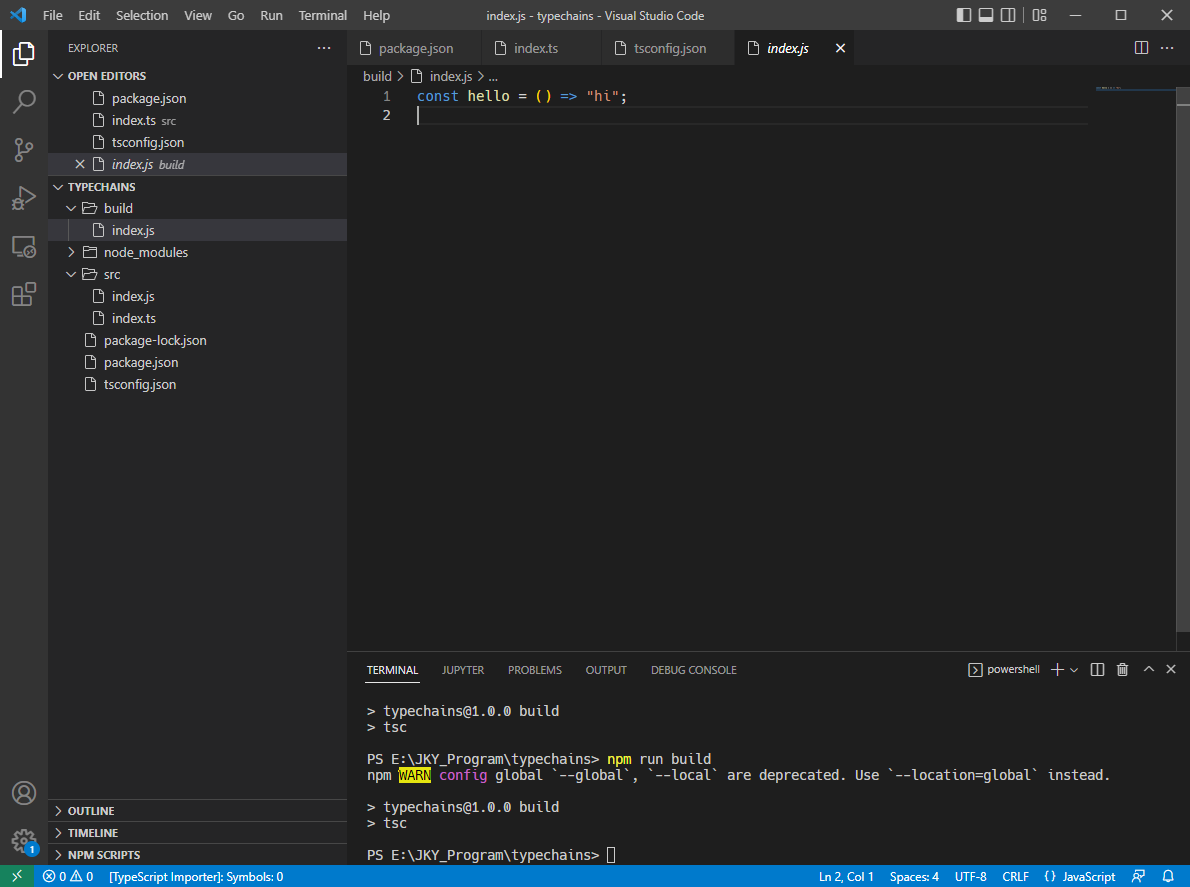
8. terminal에서 [>npm run build] 해서 컴파일 해준다.
해주면 [>tsc]가 나오는 걸 확인할 수 있고,
build 폴더에 index.js 파일이 생성됨.
[index.ts]
class Black {
constructor (private data:string) {}
static hello() {
return "hi";
}
}
[index.js]
class Black {
constructor(data) {
this.data = data;
}
static hello() {
return "hi";
}
}
#5.2 Lib Configuration
lib는 합쳐진 라이브러리의 정의 파일(declaration file)을 특정해주는 역할.
js file이 어떤 환경에서 사용될지. target runtime environment.
"lib": ["ES6", "DOM"]
=> ES6를 지원하는 서버와, DOM, 즉 브라우저 환경에서 코드를 실행시킬꺼얌.
DOM을 추가해주고, ts file에서 document를 사용하면, ts는 document가 뭔지 암.
document가 갖고 있는 모든 이벤트와 메소드를 쓸 수 있음.
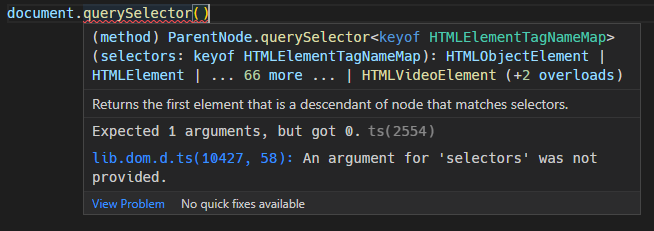
위처럼 ts는 모든 것의 타입을 설명해주고 있음.
Ctrl키를 누르고 메소드 클릭하면 lib.dom.d.ts 파일로 이동함.
위 설명에 링크를 클릭해도 됨.
node_modules/typescript/lib 폴더 안에 있음.
ts는 브라우저의 API와 타입을 알고 있기 때문에 굉장히 편리함.
lib에서 정의해줬기 때문에 자동완성 기능을 사용할 수 있음.
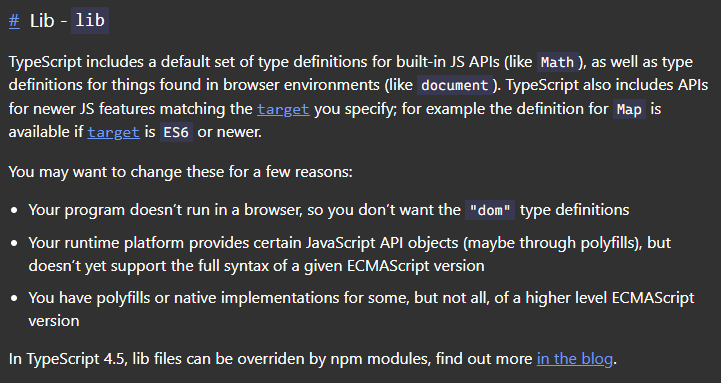
#5.3 Declaration Files
보통 js로 만들어진 패키지와 라이브러리를 사용하는데,
ts에게 그 js 파일의 모양을 설명해줘야함. (js 타입을 설명해줘야함.)
myPackage.js 파일을 만들어서 index.ts에서 써보자.
[myPackage.js]
export function init(config) {
return true;
}
export function exit(code) {
return code + 1;
}
tsconfig.json 컴파일 옵션에
"strict": true
도 추가해줌.
declaration file : js code의 모양을 ts에 설명해주는 파일 (.d.ts) (like lib.dom.d.ts)
-
myPackage.js의 function을 사용하기 위해서 다음과 같이 해줘야 함.
myPackage가 node_modules에 들어있는 것이라고 가정하고,
myPackage.d.ts 파일을 만들어 줌. (js의 타입을 알려주는 것)
interface Config {
url:string
}
declare module "myPackage" {
function init(config:Config):boolean;
function exit(code:number):number;
}
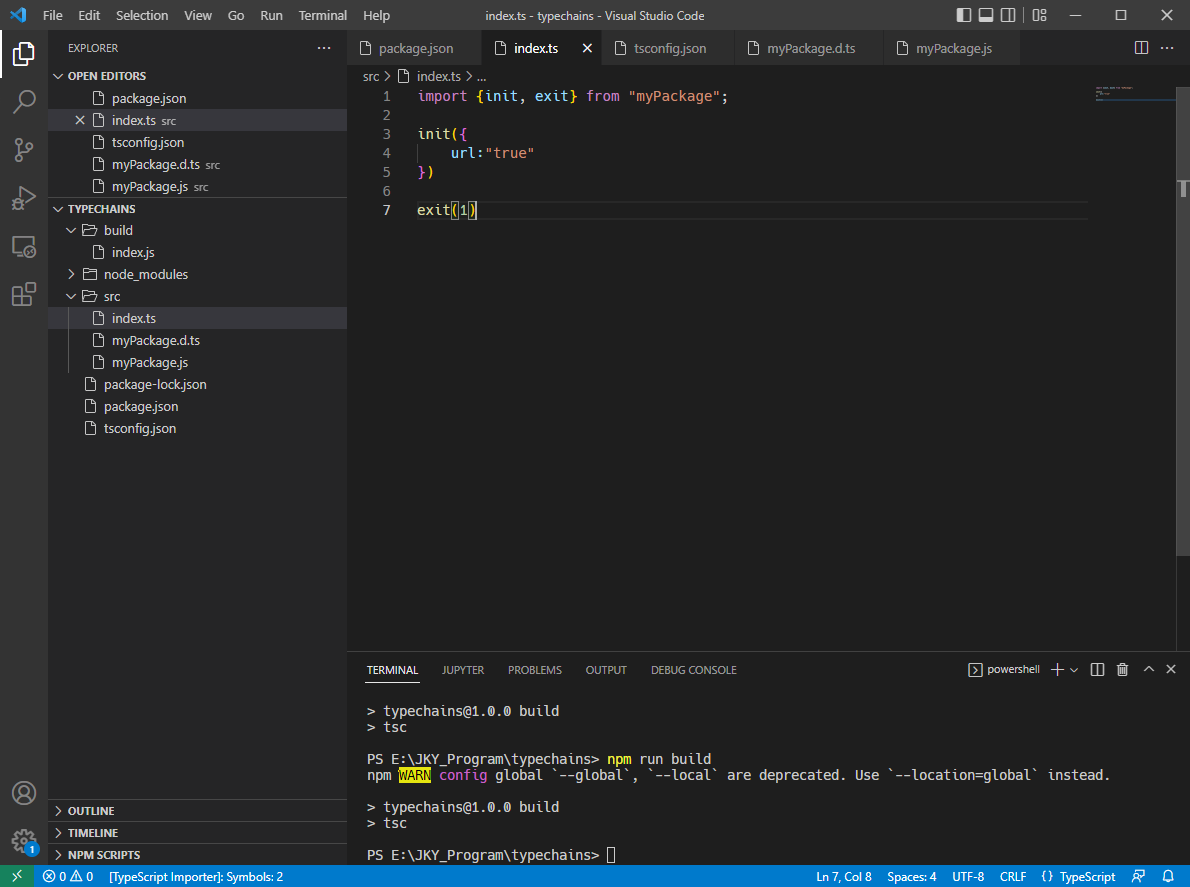
#5.4 JSDoc
d.ts 파일을 쓰지 않고, js 파일을 ts에서 쓰는 방법
=> tsconfig.json 에서 컴파일 옵션에
"allowJs": true
이거를 추가해주고,
index.ts 파일에서
import {init, exit} from "./myPackage";
init({
url:"true"
})
exit(1)
이렇게 써줌
-
js 파일을 그대로 쓰고 싶은데, ts 보호를 받고 싶으면 => JSDoc 사용.
myPackage.js
// @ts-check
// ts가 아래 comment를 읽고 type을 체크함
/**
* Initializes the project
* @param {object} config
* @param {boolean} config.debug
* @param {string} config.url
* @returns boolean
*/
export function init(config) {
return true;
}
/**
*
* @param {number} code
* @returns number
*/
export function exit(code) {
return code + 1;
}
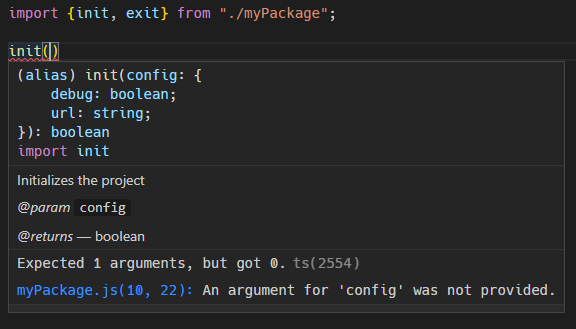
다음강의 보러가기.
2022.06.17 - [IT] - [Nomad Coders] TypeScript로 블록체인 만들기 #5.5 to #5.8 정리 (완강)
[Nomad Coders] TypeScript로 블록체인 만들기 #5.5 to #5.8 정리 (완강)
이전강의 보러가기. #5.5 Blocks VSCode 추가로 환경설정 하기 => ts-node, nodemon 설치하기 package.json "scripts"에 아래와 같이 추가. "dev": "ts-node src/index", "start": "node build/index.js" (확장자..
ella-devblog.tistory.com
'IT > DEV Study' 카테고리의 다른 글
[TypeScript] 작은 따옴표(')와 백틱(`)을 구분 못한 바보의 한탄 TT (421) | 2022.07.11 |
---|---|
[Nomad Coders] TypeScript로 블록체인 만들기 #5.5 to #5.8 정리 (완강) (0) | 2022.06.17 |
[Nomad Coders] TypeScript로 블록체인 만들기 #4 정리 (0) | 2022.06.16 |
[Nomad Coders] TypeScript로 블록체인 만들기 #3 정리 (0) | 2022.06.15 |
[Nomad Coders] TypeScript로 블록체인 만들기 #1, #2 정리 (0) | 2022.06.15 |